Local REST API 自动化接口
最后更新于:2024-02-18 11:07:58
一、简介
浏览器自动化允许您在拉力猫指纹浏览器配置文件中自动执行任务,支持Local API接口功能,通过程序化方式,读写账号配置信息、启动和关闭浏览器、查询账号列表、配置代理IP等。
API 接口中文版本 文档详见:拉力猫 REST API 接口文档
二、拉力猫指纹浏览器自动化基于Selenium WebDriver,同时也支持Puppeteer等自动化框架
通常情况下,如果您运行Selenium代码,首先将连接到Chrome驱动,然后设置您所需要的功能。而将拉力猫与Selenium代码结合使用时,您无需这样操作。您将使用Web Driver程序,通过本地端口连接到拉力猫应用或某浏览器配置文件,设置所需功能,在预定义的浏览器配置文件中执行Selenium命令。
当然你也可以脱离 Selenium 和 Puppeteer 自动化框架,可以直接调用我们的API来执行自动化操作。
三、支持所有语言编写:
拉力猫API支持任何语言,本教程的最下面有Python和Java的案例。
四、在 拉力猫浏览器 中使用:
1、定义拉力猫客户端地址和端口
您需要提前定义软件端口以使用自动化。以下是定义端口的方法:
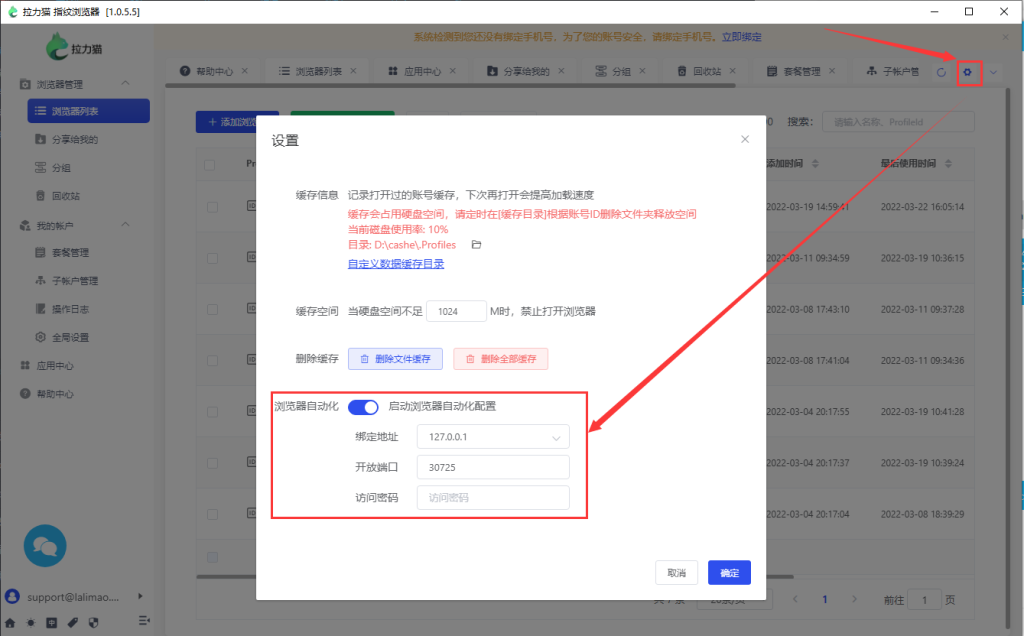
在软件界面右上右上角的【设置】按钮图标,进入后打开【启用浏览器自动化设置】,并在监听端口中设置能使用端口,这里默认是30725,另外你也可以设置一个访问密码。随后,您就可以通过定义的端口连接到拉力猫指纹浏览器了。
2、绑定服务器API令牌
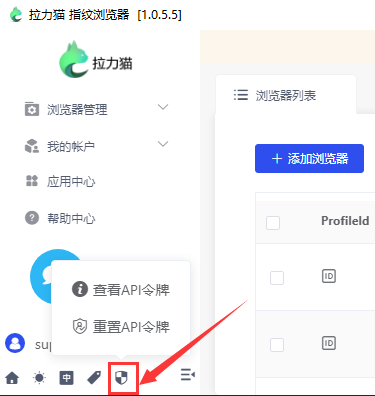
3、注意一下拉力猫指纹浏览器内核版本:
如果是96,就下载Python
http://chromedriver.storage.googleapis.com/96.0.4664.45/chromedriver_win32.zip
如果是98,就下载Python
http://chromedriver.storage.googleapis.com/98.0.4758.80/chromedriver_win32.zip
chromedriver 版本不对应,会造成自动化失败。
如果不能关闭浏览器,可以使用 http://127.0.0.1:30725/api/v1/profile/stop?profileId=xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx 接口来关闭指定配置的浏览器进程。
如果你运行代码只能打开拉力猫浏览器,并没有打开网站,那可能要把chromedriver.exe复制到你的 python 安装目录里了,还有一种可能是你选了移动仿真模式。
4、接口还可以传入代理服务器信息,如果传入代理信息会覆盖配置文件里的代理信息,这种覆盖是临时性的,不会真的修改配置文件,只对自动化接口有效:Markup
http://127.0.0.1:30725/api/v1/profile/start?automation=true&profileId=xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx&proxytype=socks5&proxyserver=ip&proxyport=1080&proxyusername=&proxypassword=
C++
代理类型可能是这4种:
proxytype=socks5
proxytype=socks4
proxytype=http
proxytype=https
代理用户名和密码可以不传为空。
五、Python 案例:
from selenium import webdriver
from selenium.webdriver.chrome.options import Options
import requests
mla_profile_id = 'xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx'
mla_url = 'http://127.0.0.1:30725/api/v1/profile/start?automation=true&profileId='+mla_profile_id
resp = requests.get(mla_url)
json = resp.json()
print(json['value'])
chrome_options = Options()
chrome_options.add_experimental_option("debuggerAddress", json['value'][7:])
chrome_driver = r"chromedriver.exe"
#http://chromedriver.storage.googleapis.com/79.0.3945.36/chromedriver_win32.zip
#下载 chromedriver 文件放到python目录
driver = webdriver.Chrome(chrome_driver, chrome_options=chrome_options)
driver.get('https://www.lalimao.com/')
executor_url = driver.command_executor._url
session_id = driver.session_id
print(executor_url)
print(session_id)
print('ok it is done')
driver.quit()
六、JAVA 案例:
package com.ruoyi.common.spider.reptile;
import cn.hutool.json.JSONObject;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.chrome.ChromeOptions;
import java.io.BufferedReader;
import java.io.InputStreamReader;
import java.net.HttpURLConnection;
import java.net.URL;
/**
* @author lalimao
* <dependency>
* <groupId>org.seleniumhq.selenium</groupId>
* <artifactId>selenium-java</artifactId>
* <version>3.141.59</version>
* </dependency>
*/
public class ProductChrome {
public static void main(String[] args) throws Exception {
ProductChrome pc = new ProductChrome();
String profileId = UUID.randomUUID().toString();
//根据profileId打开并获取远程调试地址
URL url = new URL(pc.startProfile("xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx"));
//使用远程调试地址连接到打开的chrome浏览器
System.setProperty("webdriver.chrome.driver", "D:\\lalimao\\chrome\\90.0.4430.85\\chromedriver.exe");//指到驱动位置
ChromeOptions chromeOptions = new ChromeOptions();
chromeOptions.setExperimentalOption("debuggerAddress", url.getAuthority());
WebDriver driver = new ChromeDriver(chromeOptions);
//访问lalimao
driver.get("https://www.lalimao.com/");
System.out.println(driver.getTitle());
driver.quit();
}
private String startProfile(String profileId) throws Exception {
String url = "http://127.0.0.1:30725/api/v1/profile/start?automation=true&profileId=" + profileId;
URL obj = new URL(url);
HttpURLConnection con = (HttpURLConnection) obj.openConnection();
con.setRequestMethod("GET");
BufferedReader in = new BufferedReader(
new InputStreamReader(con.getInputStream()));
String inputLine;
StringBuffer response = new StringBuffer();
while ((inputLine = in.readLine()) != null) {
response.append(inputLine);
}
in.close();
JSONObject jsonResponse = new JSONObject(response.toString());
return jsonResponse.getStr("value");
}
}